mirror of
https://github.com/go-gitea/gitea
synced 2024-06-01 00:45:46 +00:00
Co-Author: @silverwind @wxiaoguang Replace: #24404 See: - [defining configuration variables for multiple workflows](https://docs.github.com/en/actions/learn-github-actions/variables#defining-configuration-variables-for-multiple-workflows) - [vars context](https://docs.github.com/en/actions/learn-github-actions/contexts#vars-context) Related to: - [x] protocol: https://gitea.com/gitea/actions-proto-def/pulls/7 - [x] act_runner: https://gitea.com/gitea/act_runner/pulls/157 - [x] act: https://gitea.com/gitea/act/pulls/43 #### Screenshoot Create Variable: 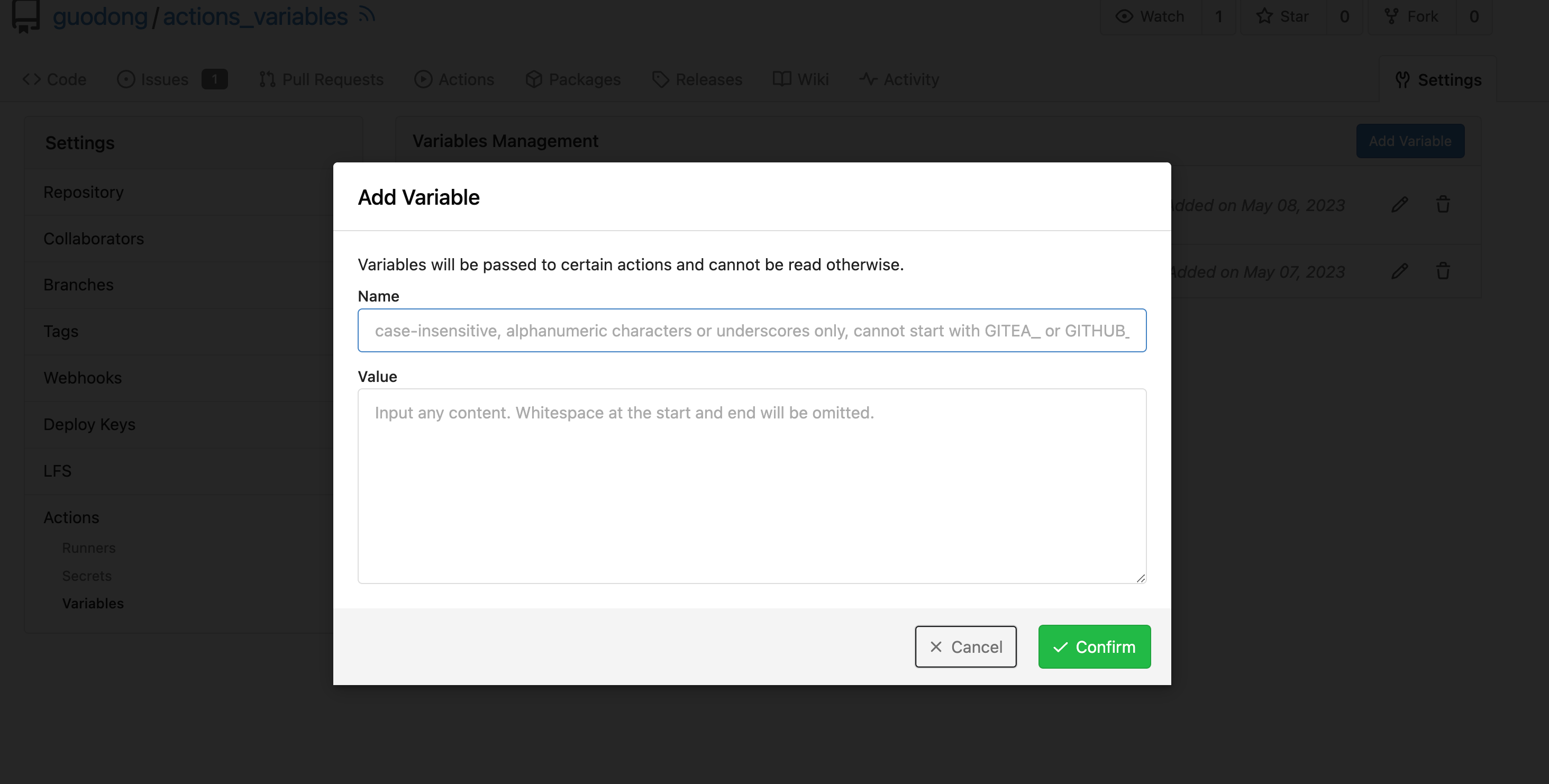 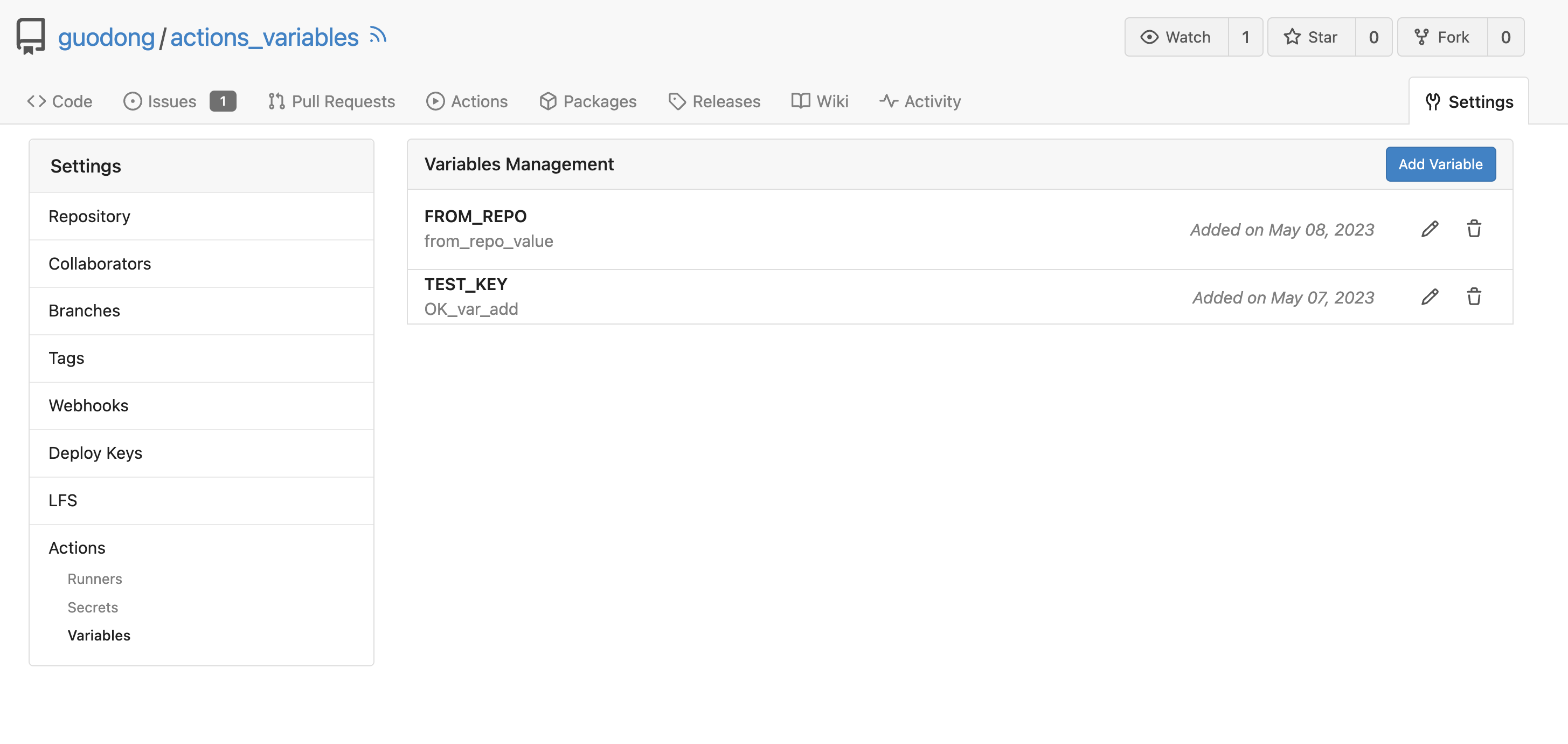 Workflow: ```yaml test_vars: runs-on: ubuntu-latest steps: - name: Print Custom Variables run: echo "${{ vars.test_key }}" - name: Try to print a non-exist var run: echo "${{ vars.NON_EXIST_VAR }}" ``` Actions Log: 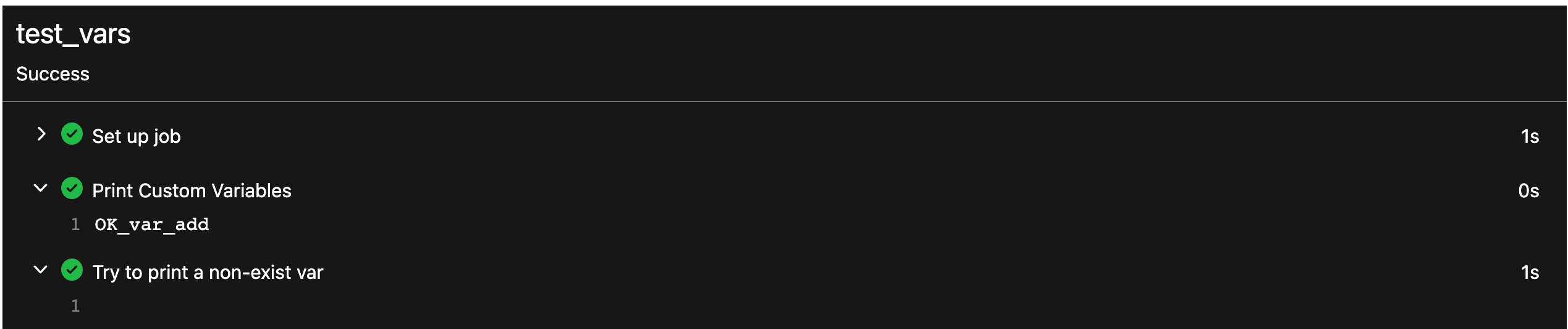 --- This PR just implement the org / user (depends on the owner of the current repository) and repo level variables, The Environment level variables have not been implemented. Because [Environment](https://docs.github.com/en/actions/deployment/targeting-different-environments/using-environments-for-deployment#about-environments) is a module separate from `Actions`. Maybe it would be better to create a new PR to do it. --------- Co-authored-by: silverwind <me@silverwind.io> Co-authored-by: wxiaoguang <wxiaoguang@gmail.com> Co-authored-by: Giteabot <teabot@gitea.io>
120 lines
2.8 KiB
Go
120 lines
2.8 KiB
Go
// Copyright 2023 The Gitea Authors. All rights reserved.
|
|
// SPDX-License-Identifier: MIT
|
|
|
|
package setting
|
|
|
|
import (
|
|
"errors"
|
|
"net/http"
|
|
|
|
"code.gitea.io/gitea/modules/base"
|
|
"code.gitea.io/gitea/modules/context"
|
|
"code.gitea.io/gitea/modules/setting"
|
|
shared "code.gitea.io/gitea/routers/web/shared/actions"
|
|
)
|
|
|
|
const (
|
|
tplRepoVariables base.TplName = "repo/settings/actions"
|
|
tplOrgVariables base.TplName = "org/settings/actions"
|
|
tplUserVariables base.TplName = "user/settings/actions"
|
|
)
|
|
|
|
type variablesCtx struct {
|
|
OwnerID int64
|
|
RepoID int64
|
|
IsRepo bool
|
|
IsOrg bool
|
|
IsUser bool
|
|
VariablesTemplate base.TplName
|
|
RedirectLink string
|
|
}
|
|
|
|
func getVariablesCtx(ctx *context.Context) (*variablesCtx, error) {
|
|
if ctx.Data["PageIsRepoSettings"] == true {
|
|
return &variablesCtx{
|
|
RepoID: ctx.Repo.Repository.ID,
|
|
IsRepo: true,
|
|
VariablesTemplate: tplRepoVariables,
|
|
RedirectLink: ctx.Repo.RepoLink + "/settings/actions/variables",
|
|
}, nil
|
|
}
|
|
|
|
if ctx.Data["PageIsOrgSettings"] == true {
|
|
return &variablesCtx{
|
|
OwnerID: ctx.ContextUser.ID,
|
|
IsOrg: true,
|
|
VariablesTemplate: tplOrgVariables,
|
|
RedirectLink: ctx.Org.OrgLink + "/settings/actions/variables",
|
|
}, nil
|
|
}
|
|
|
|
if ctx.Data["PageIsUserSettings"] == true {
|
|
return &variablesCtx{
|
|
OwnerID: ctx.Doer.ID,
|
|
IsUser: true,
|
|
VariablesTemplate: tplUserVariables,
|
|
RedirectLink: setting.AppSubURL + "/user/settings/actions/variables",
|
|
}, nil
|
|
}
|
|
|
|
return nil, errors.New("unable to set Variables context")
|
|
}
|
|
|
|
func Variables(ctx *context.Context) {
|
|
ctx.Data["Title"] = ctx.Tr("actions.variables")
|
|
ctx.Data["PageType"] = "variables"
|
|
ctx.Data["PageIsSharedSettingsVariables"] = true
|
|
|
|
vCtx, err := getVariablesCtx(ctx)
|
|
if err != nil {
|
|
ctx.ServerError("getVariablesCtx", err)
|
|
return
|
|
}
|
|
|
|
shared.SetVariablesContext(ctx, vCtx.OwnerID, vCtx.RepoID)
|
|
if ctx.Written() {
|
|
return
|
|
}
|
|
|
|
ctx.HTML(http.StatusOK, vCtx.VariablesTemplate)
|
|
}
|
|
|
|
func VariableCreate(ctx *context.Context) {
|
|
vCtx, err := getVariablesCtx(ctx)
|
|
if err != nil {
|
|
ctx.ServerError("getVariablesCtx", err)
|
|
return
|
|
}
|
|
|
|
if ctx.HasError() { // form binding validation error
|
|
ctx.JSONError(ctx.GetErrMsg())
|
|
return
|
|
}
|
|
|
|
shared.CreateVariable(ctx, vCtx.OwnerID, vCtx.RepoID, vCtx.RedirectLink)
|
|
}
|
|
|
|
func VariableUpdate(ctx *context.Context) {
|
|
vCtx, err := getVariablesCtx(ctx)
|
|
if err != nil {
|
|
ctx.ServerError("getVariablesCtx", err)
|
|
return
|
|
}
|
|
|
|
if ctx.HasError() { // form binding validation error
|
|
ctx.JSONError(ctx.GetErrMsg())
|
|
return
|
|
}
|
|
|
|
shared.UpdateVariable(ctx, vCtx.RedirectLink)
|
|
}
|
|
|
|
func VariableDelete(ctx *context.Context) {
|
|
vCtx, err := getVariablesCtx(ctx)
|
|
if err != nil {
|
|
ctx.ServerError("getVariablesCtx", err)
|
|
return
|
|
}
|
|
shared.DeleteVariable(ctx, vCtx.RedirectLink)
|
|
}
|